12 Python Tips For Beginner Success
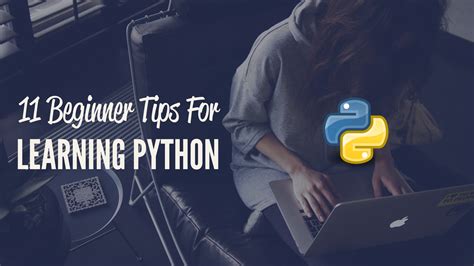
Python is a popular and versatile programming language that has become a staple in the world of computer science and data analysis. As a beginner, it can be overwhelming to navigate the vast amount of resources and tutorials available. However, with the right guidance and tips, anyone can quickly get started with Python and achieve success. In this article, we will cover 12 essential Python tips for beginner success, including best practices, common pitfalls to avoid, and expert advice from seasoned developers.
Setting Up Your Environment
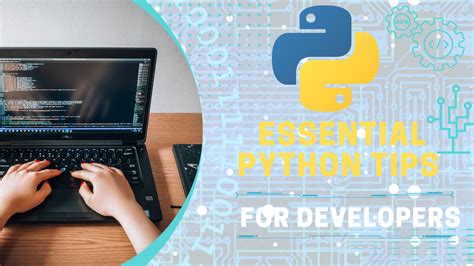
Before diving into the world of Python, it’s essential to set up your environment correctly. This includes installing the latest version of Python, choosing a suitable IDE (Integrated Development Environment), and familiarizing yourself with the basics of the language. Python 3.x is the recommended version for beginners, as it offers the most up-to-date features and security patches. Some popular IDEs for Python include PyCharm, Visual Studio Code, and Sublime Text.
Choosing the Right IDE
When choosing an IDE, consider the following factors: ease of use, features, and compatibility. PyCharm is a popular choice among beginners, as it offers a free community edition and a user-friendly interface. Visual Studio Code is another excellent option, as it provides a lightweight and customizable environment. Ultimately, the choice of IDE depends on personal preference and the specific needs of your project.
IDE | Features | Compatibility |
---|---|---|
PyCharm | Code completion, debugging, project management | Windows, macOS, Linux |
Visual Studio Code | Code completion, debugging, extensions | Windows, macOS, Linux |
Sublime Text | Code completion, debugging, customization | Windows, macOS, Linux |

Basic Syntax and Data Types
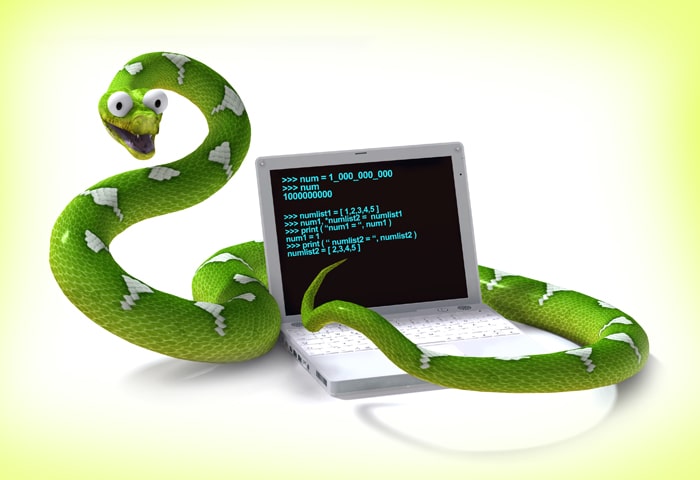
Once you have your environment set up, it’s time to learn the basics of Python syntax and data types. Python is known for its simplicity and readability, making it an excellent language for beginners. Some essential data types in Python include integers, floats, strings, and lists. Understanding these data types is crucial for writing effective and efficient code.
Working with Variables
In Python, variables are used to store and manipulate data. When working with variables, it’s essential to understand the concepts of assignment and scoping. Assignment refers to the process of assigning a value to a variable, while scoping refers to the region of the code where a variable is accessible. Understanding these concepts will help you write clean and organized code.
- Use meaningful variable names to improve code readability
- Avoid using reserved keywords as variable names
- Understand the difference between local and global variables
Control Structures and Functions
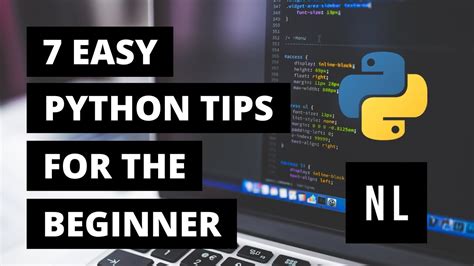
Control structures and functions are essential components of any programming language. In Python, control structures include if-else statements, for loops, and while loops. Functions, on the other hand, are reusable blocks of code that take arguments and return values. Understanding these concepts will help you write efficient and modular code.
Working with Functions
When working with functions, it’s essential to understand the concepts of arguments and return values. Arguments refer to the values passed to a function, while return values refer to the values returned by a function. Understanding these concepts will help you write effective and reusable code.
Function | Arguments | Return Value |
---|---|---|
print() | string or variable | None |
len() | string or list | integer |
sum() | list of numbers | integer |
Object-Oriented Programming
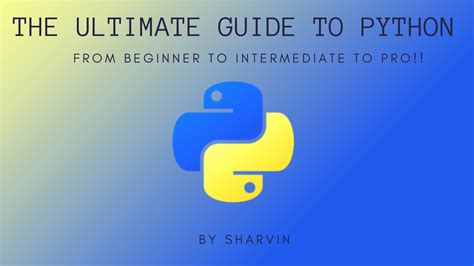
Object-Oriented Programming (OOP) is a fundamental concept in programming that involves organizing code into objects and classes. In Python, OOP is used to create reusable and modular code. Some essential concepts in OOP include classes, objects, inheritance, and polymorphism.
Working with Classes
When working with classes, it’s essential to understand the concepts of attributes and methods. Attributes refer to the data members of a class, while methods refer to the functions that operate on that data. Understanding these concepts will help you write effective and reusable code.
- Use classes to organize related data and behavior
- Understand the difference between instance and class attributes
- Use inheritance to create a hierarchy of classes
Debugging and Error Handling

Debugging and error handling are essential skills for any programmer. In Python, debugging involves using tools such as print statements and debuggers to identify and fix errors. Error handling, on the other hand, involves using try-except blocks to catch and handle exceptions.
Working with Exceptions
When working with exceptions, it’s essential to understand the concepts of try and except blocks. Try blocks contain the code that may raise an exception, while except blocks contain the code that handles the exception. Understanding these concepts will help you write robust and reliable code.
Exception | Description |
---|---|
SyntaxError | Invalid syntax |
NameError | Undefined variable |
TypeError | Invalid data type |
Best Practices and Coding Standards

Best practices and coding standards are essential for writing clean, readable, and maintainable code. In Python, some essential best practices include using meaningful variable names, following PEP 8 guidelines, and using comments to document code.
Working with Comments
When working with comments, it’s essential to understand the concepts of inline comments and block comments. Inline comments are used to explain a single line of code, while block comments are used to explain a block of code. Understanding these concepts will help you write clean and readable code.
- Use comments to document your code
- Follow PEP 8 guidelines for coding style
- Use meaningful variable names to improve code readability
What is the best way to learn Python?
+The best way to learn Python is by practicing and working on real-world projects. Start with the basics and gradually move on to more advanced topics. Use online resources such as tutorials, videos, and documentation to learn and improve your skills.
What are the most common mistakes made by Python beginners?
+Some common mistakes made by Python beginners include using