Cse 365 Solutions

CSE 365 is a course that focuses on the design and analysis of algorithms, which are the building blocks of computer science. The course provides a comprehensive introduction to the field of algorithms, including the basics of algorithm design, analysis, and implementation. In this article, we will provide solutions to some of the common problems and topics covered in CSE 365.
Introduction to Algorithms

Algorithms are step-by-step procedures for solving problems or performing tasks. They are essential in computer science, as they enable computers to process and analyze large amounts of data efficiently. There are several types of algorithms, including sorting algorithms, searching algorithms, and graph algorithms. Each type of algorithm has its own strengths and weaknesses, and the choice of algorithm depends on the specific problem being solved.
Time and Space Complexity
When analyzing algorithms, it is essential to consider their time complexity and space complexity. Time complexity refers to the amount of time an algorithm takes to complete, while space complexity refers to the amount of memory it requires. The time and space complexity of an algorithm can be expressed using Big O notation, which provides an upper bound on the complexity of the algorithm. For example, an algorithm with a time complexity of O(n) takes linear time, while an algorithm with a time complexity of O(n^2) takes quadratic time.
Algorithm | Time Complexity | Space Complexity |
---|---|---|
Bubble Sort | O(n^2) | O(1) |
Quick Sort | O(n log n) | O(log n) |
Binary Search | O(log n) | O(1) |

Sorting Algorithms
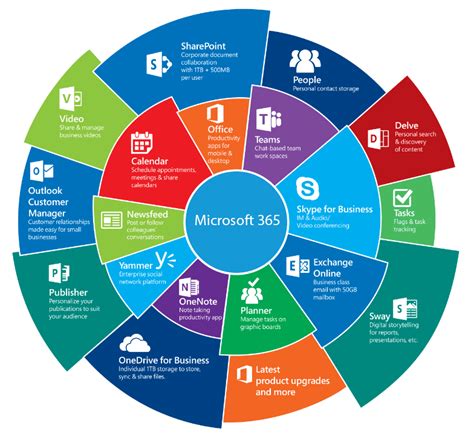
Sorting algorithms are used to arrange data in a specific order, such as ascending or descending order. There are several types of sorting algorithms, including bubble sort, selection sort, and merge sort. Each type of sorting algorithm has its own strengths and weaknesses, and the choice of algorithm depends on the specific problem being solved.
Comparison of Sorting Algorithms
The following table compares the time and space complexity of several sorting algorithms:
Algorithm | Best-Case Time Complexity | Worst-Case Time Complexity | Space Complexity |
---|---|---|---|
Bubble Sort | O(n) | O(n^2) | O(1) |
Selection Sort | O(n^2) | O(n^2) | O(1) |
Merge Sort | O(n log n) | O(n log n) | O(n) |
Searching Algorithms

Searching algorithms are used to find specific data within a larger dataset. There are several types of searching algorithms, including linear search and binary search. Each type of searching algorithm has its own strengths and weaknesses, and the choice of algorithm depends on the specific problem being solved.
Binary Search
Binary search is a fast and efficient searching algorithm that works by dividing the dataset in half and searching for the target data in one of the two halves. The following is an example of a binary search algorithm:
def binary_search(arr, target):
low = 0
high = len(arr) - 1
while low <= high:
mid = (low + high) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
low = mid + 1
else:
high = mid - 1
return -1
What is the time complexity of binary search?
+The time complexity of binary search is O(log n), making it a fast and efficient searching algorithm.
What is the space complexity of merge sort?
+The space complexity of merge sort is O(n), making it a relatively space-efficient sorting algorithm.
In conclusion, CSE 365 provides a comprehensive introduction to the design and analysis of algorithms, including the basics of algorithm design, analysis, and implementation. By understanding the different types of algorithms, including sorting and searching algorithms, and their time and space complexity, students can develop the skills and knowledge needed to solve complex problems in computer science.