How Swift Measures Time Accurately? Easy Tips

Measuring time accurately is crucial in various aspects of programming, including game development, scientific simulations, and real-time systems. Swift, being a modern and powerful programming language, provides several ways to measure time accurately. In this article, we will delve into the details of how Swift measures time and provide easy tips for accurate time measurement.
Introduction to Swift’s Time Measurement
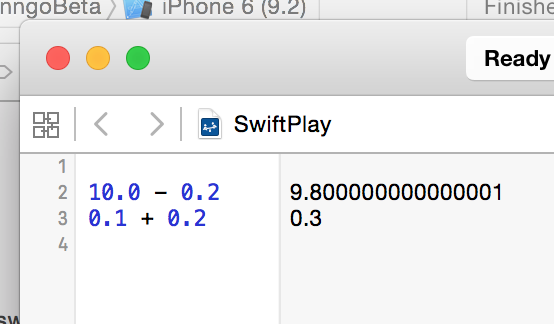
Swift provides several classes and functions for measuring time, including Date, DateComponents, and DispatchTime. These classes and functions allow developers to measure time with high precision, making them suitable for a wide range of applications. The Date class, for example, represents a point in time, while the DateComponents class represents the components of a date, such as year, month, and day.
Using Date and DateComponents
The Date class is a fundamental class in Swift for measuring time. It provides several initializers and methods for creating and manipulating dates. The DateComponents class, on the other hand, provides a way to represent the components of a date. By using these classes together, developers can measure time accurately and perform various date-related calculations. For example, you can use the Date class to get the current date and time, and then use the DateComponents class to extract the year, month, and day components.
Class/Function | Description |
---|---|
Date | Represents a point in time |
DateComponents | Represents the components of a date |
DispatchTime | Represents a point in time relative to the dispatcher |

Measuring Time with DispatchTime
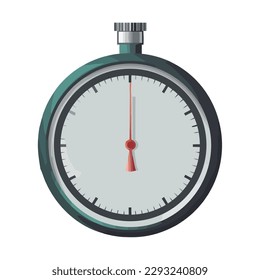
The DispatchTime class provides a way to measure time relative to the dispatcher. It’s commonly used in concurrent programming and provides high precision time measurement. The DispatchTime class has several initializers and methods for creating and manipulating dispatch times. For example, you can use the DispatchTime.now() function to get the current dispatch time, and then use the DispatchTime.uptimeNanoseconds property to get the uptime in nanoseconds.
Converting Between Date and DispatchTime
Swift provides several functions for converting between Date and DispatchTime. The Date.timeIntervalSinceReferenceDate property, for example, returns the time interval between the date and the reference date. You can use this property to convert a Date object to a DispatchTime object. Similarly, you can use the DispatchTime.now().uptimeNanoseconds property to convert a DispatchTime object to a Date object.
Here's an example of how to convert between Date and DispatchTime: ```swift let date = Date() let dispatchTime = DispatchTime.now() let timeInterval = date.timeIntervalSinceReferenceDate let uptimeNanoseconds = dispatchTime.uptimeNanoseconds ```
What is the difference between Date and DispatchTime?
+The Date class represents a point in time, while the DispatchTime class represents a point in time relative to the dispatcher. The DispatchTime class provides higher precision time measurement and is commonly used in concurrent programming.
How do I convert between Date and DispatchTime?
+You can use the Date.timeIntervalSinceReferenceDate property to convert a Date object to a DispatchTime object, and the DispatchTime.now().uptimeNanoseconds property to convert a DispatchTime object to a Date object.
Best Practices for Measuring Time in Swift

When measuring time in Swift, it’s essential to follow best practices to ensure accurate and precise time measurement. Here are some tips:
- Use the Date class for measuring time in most cases, as it provides a high level of precision and is easy to use.
- Use the DispatchTime class when high precision time measurement is required, such as in concurrent programming.
- Consider the timezone and calendar used when measuring time, as it can affect the accuracy of the measurement.
- Use the DispatchTime.now().uptimeNanoseconds property to get the uptime in nanoseconds, which provides high precision time measurement.