Stanford C++ Library
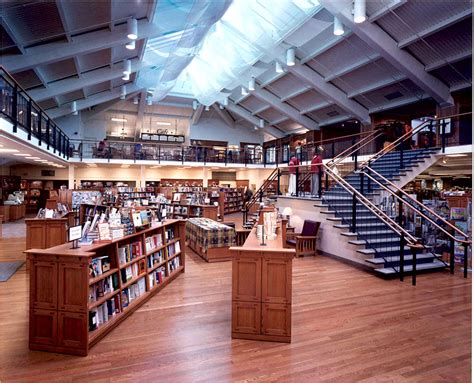
The Stanford C++ Library, also known as the Stanford GraphBase, is a comprehensive collection of C++ software libraries and tools for a wide range of applications, including computer science education, research, and software development. Developed at Stanford University, this library provides a rich set of data structures, algorithms, and utilities that can be used to solve complex problems in various fields, such as graph theory, combinatorial algorithms, and data analysis.
Overview of the Stanford C++ Library
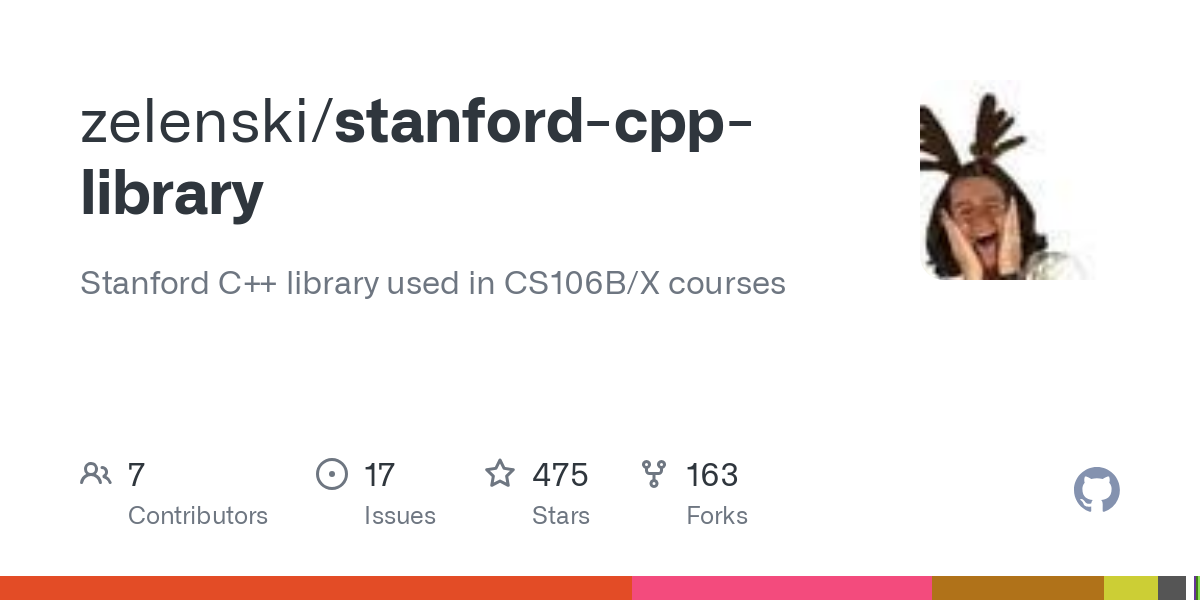
The Stanford C++ Library is designed to provide a robust and efficient platform for building a wide range of applications, from simple command-line tools to complex graphical user interfaces. The library includes a vast array of data structures, such as graphs, trees, and arrays, as well as algorithms for searching, sorting, and manipulating these data structures. Additionally, the library provides a set of utility functions for tasks such as file input/output, string manipulation, and mathematical computations.
Data Structures in the Stanford C++ Library
The Stanford C++ Library includes a variety of data structures that can be used to represent complex relationships between objects. Some of the most commonly used data structures in the library include:
- Graphs: The library provides a comprehensive implementation of graph data structures, including directed and undirected graphs, as well as algorithms for traversing and manipulating these graphs.
- Trees: The library includes a variety of tree data structures, such as binary trees, AVL trees, and red-black trees, as well as algorithms for searching, inserting, and deleting nodes in these trees.
- Arrays: The library provides a set of array data structures, including one-dimensional and multi-dimensional arrays, as well as algorithms for manipulating and searching these arrays.
Data Structure | Description |
---|---|
Graph | A non-linear data structure consisting of nodes and edges |
Tree | A hierarchical data structure consisting of nodes and edges |
Array | A linear data structure consisting of a collection of elements |

Algorithms in the Stanford C++ Library
The Stanford C++ Library includes a wide range of algorithms that can be used to solve complex problems in various fields. Some of the most commonly used algorithms in the library include:
- Searching algorithms: The library provides a set of searching algorithms, such as linear search and binary search, that can be used to find specific elements in a data structure.
- Sorting algorithms: The library includes a variety of sorting algorithms, such as bubble sort and quicksort, that can be used to arrange elements in a data structure in a specific order.
- Graph algorithms: The library provides a set of graph algorithms, such as Dijkstra’s algorithm and Bellman-Ford algorithm, that can be used to find the shortest path between nodes in a graph.
Using the Stanford C++ Library
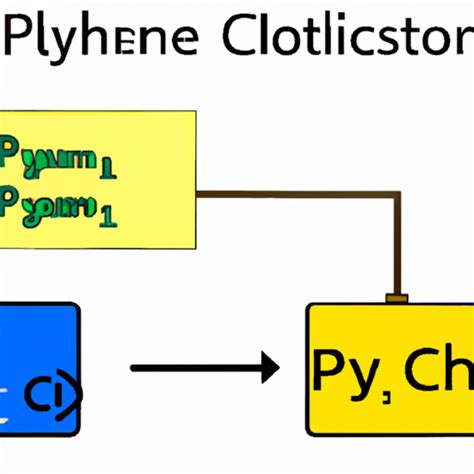
To use the Stanford C++ Library, developers can simply include the relevant header files in their C++ programs and link against the library’s object files. The library provides a comprehensive set of documentation and examples that can be used to learn how to use the various data structures and algorithms provided by the library.
Example Code
Here is an example of how to use the Stanford C++ Library to create a simple graph and perform a depth-first search:
#include <iostream>
#include <graph.h>
int main() {
// Create a new graph
Graph g;
// Add nodes to the graph
g.addNode("A");
g.addNode("B");
g.addNode("C");
// Add edges to the graph
g.addEdge("A", "B");
g.addEdge("B", "C");
g.addEdge("C", "A");
// Perform a depth-first search
g.depthFirstSearch("A");
return 0;
}
What is the Stanford C++ Library?
+The Stanford C++ Library is a comprehensive collection of C++ software libraries and tools for a wide range of applications, including computer science education, research, and software development.
What data structures are included in the Stanford C++ Library?
+The Stanford C++ Library includes a variety of data structures, such as graphs, trees, and arrays, as well as algorithms for searching, sorting, and manipulating these data structures.
How do I use the Stanford C++ Library?
+To use the Stanford C++ Library, developers can simply include the relevant header files in their C++ programs and link against the library’s object files. The library provides a comprehensive set of documentation and examples that can be used to learn how to use the various data structures and algorithms provided by the library.